Dockerizing simple Sinatra app using docker and fig
Docker is new hotness in market , docker use kernel level namespace’s and cgroups to create isolated container environment . according to Wikipedia .
UPDATE : fig is now merged in docker and now its called : compose
Docker is an open-source project that automates the deployment of applications inside software containers, by providing an additional layer of abstraction and automation of operating system–level virtualization on Linux.[Docker uses resource isolation features of the Linux kernel such as cgroups and kernel namespaces to allow independent “containers” to run within a single Linux instance, avoiding the overhead of starting virtual machines.
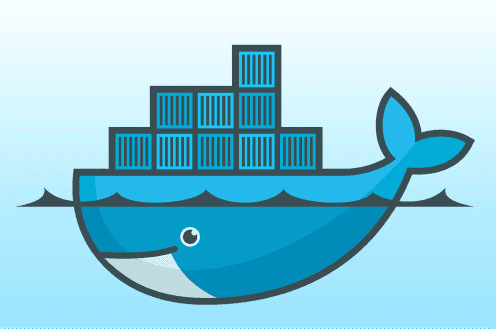
If you know what is lxc and thinking what is difference between LXC and docker then give a look at this excellent post : http://www.flockport.com/lxc-vs-docker/ .
Install Docker
lets first install docker , You can use official Documentation to get up running docker https://docs.docker.com/installation/.
$ sudo apt-get install curl
$ curl -sSL https://get.docker.com/ubuntu/ | sudo sh
and lets install fig
$ sudo apt-get install python-pip
$ sudo pip install fig
Lets test our installation
$ docker --version && fig --versions
# lets run simple hello world inside a ubuntu container
$ sudo docker run -i -t ubuntu /bin/echo "Hello from container "
Sample output
alok@ubuntu:~$ sudo docker run -i -t ubuntu /bin/echo "Hello from container "
Hello from container
give non root access for avoiding using sudo every time with docker command .
$ sudo groupadd docker
$ sudo gpasswd -a ${USER} docker
$ sudo service docker restart
okay so , I wrote this simplest sinatra app , its contains five files 2 of them related to gem file and app.rb
is our app and fig.yml
will be used as fig configuration file and Dockerfile
will be used to build our image
alok@ubuntu:~/code/ruby/app$ tree
├── app.rb
├── Dockerfile
├── fig.yml
├── Gemfile
└── Gemfile.lock
# app.rb
require "sinatra "
get '/'
"Hello World !"
end
and created Gemfille
#Gemfile
source 'https://rubygems.org'
gem 'sinatra'
okay , now lets build ruby image , I build using dockerfile because its take too much to download 800MB file .
okay . you can clone all this from github https://github.com/alokyadav15/sinatra_container.git .
#Dockerfile
FROM ubuntu
RUN apt-get update
RUN apt-get -y update
RUN apt-get -y install git git-core curl gawk g++ gcc make libc6-dev libreadline6-dev \
zlib1g-dev libssl-dev libyaml-dev libsqlite3-dev sqlite3 autoconf \
libgdbm-dev libncurses5-dev automake libtool bison pkg-config libffi-dev
RUN apt-get install -y postgresql postgresql-contrib postgresql-client libpq5 libpq-dev
RUN curl -sSL https://rvm.io/mpapis.asc | gpg --import -
RUN curl -L https://get.rvm.io | bash -s stable
ENV PATH /usr/local/rvm/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin
RUN rvm install ruby-2.1.5
RUN rvm reload
CMD source /etc/profile
CMD source /usr/local/rvm/scripts/rvm
RUN apt-get install -y nodejs npm
RUN ln -s /usr/bin/nodejs /usr/bin/node
RUN ["/bin/bash", "-l", "-c", "rvm use 2.1.5 --default" ]
RUN ["/bin/bash", "-l", "-c", "rvm requirements; gem install bundler --no-ri --no-rdoc"]
RUN ["/bin/bash", "-l", "-c", "gem list"]
RUN apt-get update -qq && apt-get install -y build-essential libpq-dev
RUN mkdir /myapp
WORKDIR /myapp
ADD Gemfile /myapp/Gemfile
RUN ["/bin/bash", "-l", "-c", "bundle install "]
fig is small utility that’s provide functionality to set up quick development environment . all it require a file fig.yml
. read more about fig on fig’s website : http://www.fig.sh/.
# fig.yml
web:
build: .
command: ["/bin/bash", "-l", "-c", "ruby app.rb"]
volumes:
- .:/myapp
ports:
- "8000:4567"
just use this Dockerfile
to build image . you can tag use tag this image to use this image for your next ruby project .
$ git clone https://github.com/alokyadav15/sinatra_container.git
$ cd sinatra_container
$ docker build -t="ubuntu/sinatra" .
$ fig build
$ fig up
it will take some time to build , it will install ruby using rvm and sinatra app .
fig up
sample output
now open your browser and point it to : http://localhost:8000 .
you will hello world program .
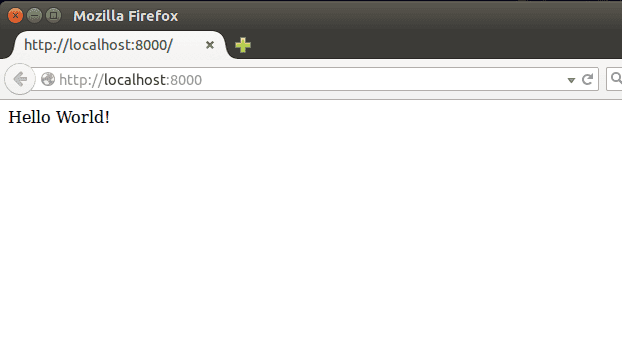
Thats all for now , thanks for reading .
Useful Links :
- Docker : https://www.docker.com/
- fig : http://www.fig.sh/
- sinatra : <www.sinatrarb.com>
- my github repo : https://github.com/alokyadav15/sinatra_container